19Django-文章列表
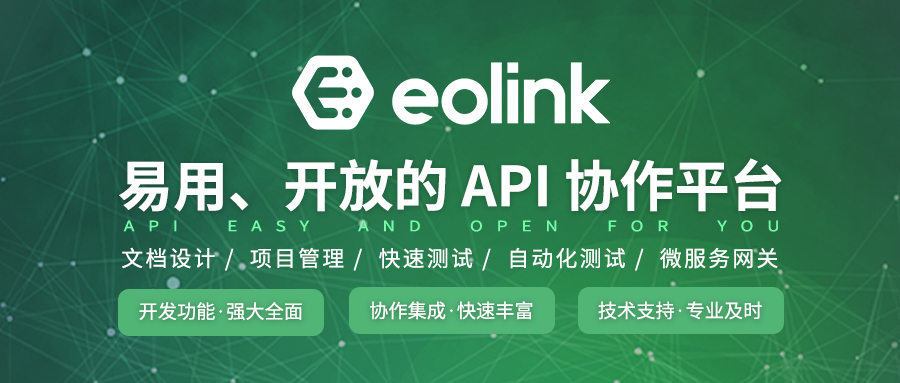
19Django-文章列表
文章列表页:
获取文章列表说到底也是从数据库查数据,因此理应先根据前端要求的格式编写一个组装数据的方法,
前端要求的数据格式:
{‘code’:200, ‘data’:{‘nickname’:’linuxTang’, ‘topics’:[{‘id’:1, ‘title’:’A’, ‘category’:’python’, ‘created_time’:’2021-12-15 21:07:20’, ‘introduce’:’AAA’, ‘author’:’qq66907360’}]}}
分析:
数据里有作者的昵称nickname,还有文章数组topics,而数组里面是一个个的字典,每一个字典就是一篇文章
又因为前端定的请求接口地址是blog_username,而这个路由正好也是我们发布文章时设置的url,所以我们就再这个路由指定的视图类中编写组装数据的方法
1开始编写组装数据的方法:
class TopicViews(View): #假设author是作者,author_topics是文章 def make_topics_res(self, author, author_topics) #外框 res = {'code':200,'data':{}} #数组框 topics_res = [] #遍历出所有的文章 for topic in author_topics: 组织数据 d = {} d['id'] = topic.id d['title'] = topic.title d['category'] = topic.category d['created_time'] = topic.created_time.strftime("%Y-%m-%d %H:%M:%S") d['introduce'] = topic.introduce d['author'] = topic.author.username #把字典追加到列表框内 topics_res.append(d) #把列表框赋值给data里的topics res['data'][topics] = topics_res #还剩下一个昵称需要赋值 res['data']['nickname'] = author.nickname return
2编写查看文章逻辑:
查看文章不需要post,所以用get方法即可,因为用户只能查看我们数据库里存在的作者文章,所以我们要对用户传过来的作者进行判断,有就展示,没有就直接return
#确认博主身份 try: author = UserProfile.objects.get(username=username) except Exception as e: result = {'code':301,'error':'作者不存在'} return
如果传过来的用户存在,我们就要用事先定义好的方法判断用户的身份,通过身份验证的用户就把他的用户名取出来和token里的用户名做对比,是本人就看自己的,不是本人就看所有作者公开的文章
#判断访客身份 visitor = get_user_by_request(request) visitor_username = None if visitor: visitor_username = visitor.username #逻辑 #如果访客的用户名等于当前博主的用户名意味着博主再访问自己的博客 if visitor_username == username: author_topics = Topic.objects.filter(author_id=username) else: #否则的话只能看公开的 author_topics = Topic.objects.filter(author_id=username,limit='public') 备注:查询某个作者的文章是一定要用author_id这个字段,因为author是文章模型类的一个外键,author_id表示文章作者的id,这个字段可以取数据库验证
经过了以上判断后,我们就获得了作者author和文章author_topics,然后调用组装数据的方法开始组装,并把组装好的数据返给前端
res = self.make_topics_res(author, author_topics) return
最后我们可能还需要对访问的内容做个判断,如果能获取到分类字段,我们就让访问者浏览分类中的内容,否则就浏览全部内容:
#判断是否查分类了 if category in ['python','linux']: #如果访客的用户名等于当前博主的用户名意味着博主再访问自己的博客 if visitor_username == username: author_topics = Topic.objects.filter(author_id=username,category=category) else: #否则的话只能看公开的 author_topics = Topic.objects.filter(author_id=username,limit='public',category=category) else: if visitor_username == username: author_topics = Topic.objects.filter(author_id=username) else: #否则的话只能看公开的 author_topics = Topic.objects.filter(author_id=username,limit='public')
最后浏览一下整个视图的代码吧:
import jsonfrom django.import JsonResponsefrom django.shortcuts import renderfrom django.views import Viewfrom django.utils.decorators import method_decoratorfrom tools.logging_dec import logging_check,get_user_by_requestfrom .models import Topicfrom user.models import UserProfile# Create your views here.#发表文章from django.forms.models import model_to_dictclass TopicViews(View): #定义一个方法返回文档里要求的字典#{‘code’:200, ‘data’:{‘nickname’:’linuxTang’, ‘topics’:[{‘id’:1, ‘title’:’A’, ‘category’:’python’, ‘created_time’:’2021-12-15 21:07:20’, ‘introduce’:’AAA’, ‘author’:’qq66907360’}]}} def make_topics_res(self,author,author_topics): res = {'code':200,'data':{}} topics_res = [] #遍历出每一篇文章 for topic in author_topics: #组织数据 #{‘id’:1, ‘title’:’A’, ‘category’:’python’, ‘created_time’:’2021-12-15 21:07:20’, ‘introduce’:’AAA’, ‘author’:’qq66907360’} d={} d['id'] = topic.id d['title'] = topic.title d['category'] = topic.category d['created_time'] = topic.create_time.strftime("%Y-%m-%d %H:%M:%S") d['introduce'] = topic.introduce d['author'] = topic.author.username #把组织好的字典添加到列表中 #[{‘id’:1, ‘title’:’A’, ‘category’:’python’, ‘created_time’:’2021-12-15 21:07:20’, ‘introduce’:’AAA’, ‘author’:’qq66907360’}] topics_res.append(d) #‘topics’:[{‘id’:1, ‘title’:’A’, ‘category’:’python’, ‘created_time’:’2021-12-15 21:07:20’, ‘introduce’:’AAA’, ‘author’:’qq66907360’}] res['data']['topics'] = topics_res ##{‘code’:200, ‘data’:{‘nickname’:’linuxTang’ res['data']['nickname'] = author.nickname print(res) return res @method_decorator(logging_check) #把自定义的方法装饰器转换成类方法可以使用的类装饰器 #文章发布 def post(self,request,username): # 拿到当前登录的用户 username = request.myuser #取出前端传过来的数据 json_str = request.body #把json串转换成字典 json_obj = json.loads(json_str) #从字典里取数据 title = json_obj.get('title') content = json_obj.get('content') content_text = json_obj.get('content_text') introduce = content_text[:30] #截取简介 limit = json_obj.get('limit') category = json_obj.get('category') #对文章权限进行判断,防止外部垃圾 if limit not in ['public','private']: result = {'code':10300,'error':'文章权限关键字非法'} return JsonResponse(result) #对文章分类做判断,也是为了安全 if category not in ['python','linux']: result = {'code': 10301, 'error': '文章分类关键字非法'} return JsonResponse(result) #创建文章发布数据 Topic.objects.create(title=title,content=content,limit=limit,category=category,introduce=introduce,author=username) return JsonResponse({'code':200}) #查看文章 def get(self,request,username): #访问博客的人分类两类,一类是访客,另外一类是博主本人,先判断传过来的用户名在数据库中是否存在 #确认博主身份 try: author = UserProfile.objects.get(username=username) #dict_author = model_to_dict(author) #dict_author['avatar'] = author.avatar.url except Exception as e: result = {'code':301,'error':'作者不存在'} return JsonResponse(result) #判断访客身份 visitor = get_user_by_request(request) visitor_username = None if visitor: visitor_username = visitor.username #温柔取值,没有返回None category = request.GET.get('category') #判断是否查分类了 if category in ['python','linux']: #如果访客的用户名等于当前博主的用户名意味着博主再访问自己的博客 if visitor_username == username: author_topics = Topic.objects.filter(author_id=username,category=category) else: #否则的话只能看公开的 author_topics = Topic.objects.filter(author_id=username,limit='public',category=category) else: if visitor_username == username: author_topics = Topic.objects.filter(author_id=username) else: #否则的话只能看公开的 author_topics = Topic.objects.filter(author_id=username,limit='public') #把博主的和查到的文章作为参数都传到响应方法make_topics_res里 #return JsonResponse({'author':dict_author,'list_arctile':[model_to_dict(i) for i in author_topics]}) res = self.make_topics_res(author, author_topics) print(res) return JsonResponse(res)#我知道原因,但不知如何解决好,我刚找了一个大神,给我弄了一下,但是请求不到数据,因为前端页面都是渲染的,所以波必须按照前端的要求写逻辑,我演示给你看一下
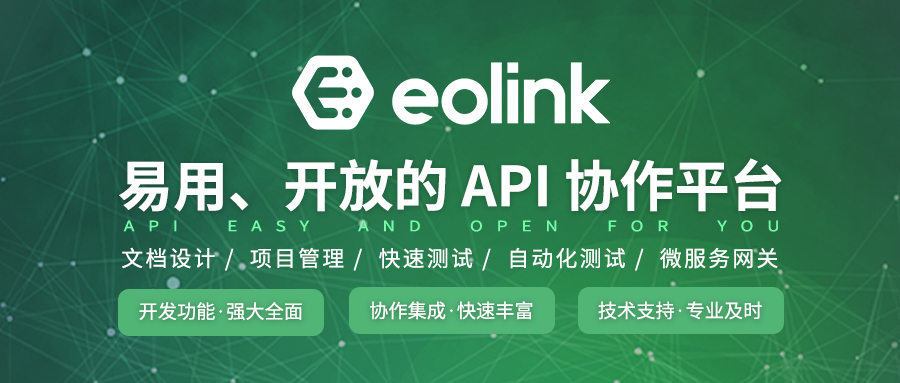
版权声明:本文内容由网络用户投稿,版权归原作者所有,本站不拥有其著作权,亦不承担相应法律责任。如果您发现本站中有涉嫌抄袭或描述失实的内容,请联系我们jiasou666@gmail.com 处理,核实后本网站将在24小时内删除侵权内容。
发表评论
暂时没有评论,来抢沙发吧~