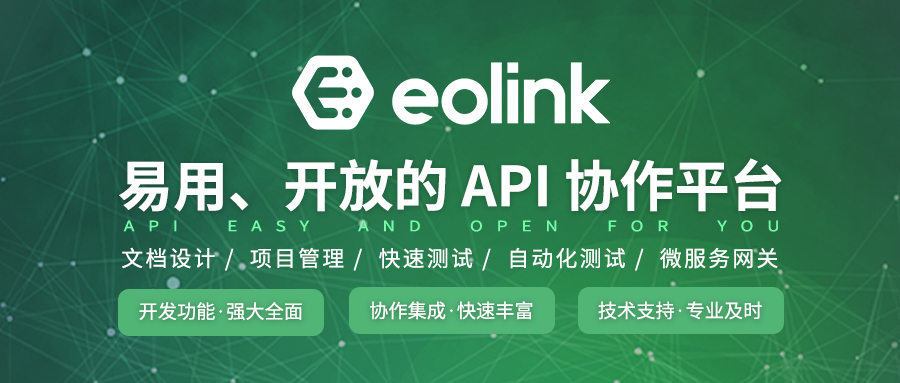
1、创建基础参数类
复制代码 代码如下:
public static class BaiduConstParams { public const string PlaceApIv2Search = "http://api.map.baidu.com/place/v2/search"; public const string PlaceApIv2Detail = "http://api.map.baidu.com/place/v2/detail"; public const string PlaceApIv2Eventsearch = "http://api.map.baidu.com/place/v2/eventsearch"; public const string PlaceApIv2Eventdetail = "http://api.map.baidu.com/place/v2/eventdetail"; public const string GeocodingApIv2 = "http://api.map.baidu.com/geocoder/v2/"; public const string GeocodingApIv2Reverse = "http://api.map.baidu.com/geocoder/v2/"; public const string TranslateApi = "http://openapi.baidu.com/public/2.0/bmt/translate"; public const string GeoconvApi = "http://api.map.baidu.com/geoconv/v1/"; }
public static class BaiduErrorMessages { public const string NotKey = "密钥不存在"; public const string LackParam = "缺少必要请求参数"; }
2、定义API错误信息与产品信息
复制代码 代码如下:
public enum BaiduLbsType { PlaceApIv2Search, PlaceApIv2Detail, PlaceApIv2Eventsearch, PlaceApIv2Eventdetail, GeocodingApIv2, GeocodingApIv2Reverse, Translate, Geoconv }
public enum Status { ///
/// 正常 /// Ok = 0, ///
/// 请求参数非法 /// ParameterInvalid = 2, ///
/// 权限校验失败 /// VerifyFailure = 3, ///
/// 配额校验失败 /// QuotaFailure = 4, ///
/// 不存在或者非法 /// AkFailure = 5, ///
/// Transform 内部错误 /// InternalError = 1, ///
/// from非法 /// FromIllegal = 21, ///
/// to非法 /// ToIllegal = 22, ///
/// coords非法 /// CoordsIllegal = 24, ///
/// coords个数非法,超过限制 /// CoordsCountIllegal = 25
}
3、定义API结果返回实体映射类
复制代码 代码如下:
public class BaiduGeocodingResults { ///
/// 返回结果状态值, 成功返回0,其他值请查看附录。 /// [JsonProperty(PropertyName = "status")] public Status Status;
///
/// 返回结果状态值, 成功返回0,其他值请查看附录。 /// [JsonProperty(PropertyName = "result")] public BaiduGeocodingResult Result; }
public class BaiduGeocodingResult { ///
/// 经纬度坐标 /// [JsonProperty(PropertyName = "location")] public BaiduGeocodingLoaction Location; ///
/// 位置的附加信息,是否精确查找。1为精确查找,0为不精确。 /// [JsonProperty(PropertyName = "precise")] public int Precise; ///
/// 可信度 /// [JsonProperty(PropertyName = "confidence")] public int Confidence; ///
/// 地址类型 /// [JsonProperty(PropertyName = "level")] public string Level;
///
/// 结构化地址信息 /// [JsonProperty(PropertyName = "formatted_address")] public string FormattedAddress;
///
/// 所在商圈信息,如 "人民大学,中关村,苏州街" /// [JsonProperty(PropertyName = "business")] public string Business;
///
/// 具体地址 /// [JsonProperty(PropertyName = "addressComponent")] public BaiduGeocodingAddress AddressComponent; }
public class BaiduGeocodingLoaction { ///
/// 纬度值 /// [JsonProperty(PropertyName = "lat")] public decimal Lat; ///
/// 经度值 /// [JsonProperty(PropertyName = "lng")] public decimal Lng; }
public class BaiduGeocodingAddress { ///
/// 城市名 /// [JsonProperty(PropertyName = "city")] public string City; ///
/// 区县名 /// [JsonProperty(PropertyName = "district")] public string District; ///
/// 省名 /// [JsonProperty(PropertyName = "province")] public string Province; ///
/// 街道名 /// [JsonProperty(PropertyName = "street")] public string Street; ///
/// 街道门牌号 /// [JsonProperty(PropertyName = "street_number")] public string StreetNumber; }
4、创建API通用处理类
复制代码 代码如下:
public class BaiduLbs { private readonly string _key;
public static string CurrentRequest = "";
public BaiduLbs(string key) { _key = key; }
///
/// 请求 /// ///
///
///
///
public void Request(string param, BaiduLbsType baiduLbsType, Encoding encoding, Action
action) { WebClient webClient = new WebClient { Encoding = encoding }; string url = ""; switch (baiduLbsType) { case BaiduLbsType.PlaceApIv2Search: url = string.Format(BaiduConstParams.PlaceApIv2Search + "?{0}", param); break; case BaiduLbsType.PlaceApIv2Detail: url = string.Format(BaiduConstParams.PlaceApIv2Detail + "?{0}", param); break; case BaiduLbsType.PlaceApIv2Eventsearch: url = string.Format(BaiduConstParams.PlaceApIv2Eventsearch + "?{0}", param); break; case BaiduLbsType.PlaceApIv2Eventdetail: url = string.Format(BaiduConstParams.PlaceApIv2Eventdetail + "?{0}", param); break; case BaiduLbsType.GeocodingApIv2: case BaiduLbsType.GeocodingApIv2Reverse: url = string.Format(BaiduConstParams.GeocodingApIv2 + "?{0}", param); break; case BaiduLbsType.Translate: url = string.Format(BaiduConstParams.TranslateApi + "?{0}", param); break; case BaiduLbsType.Geoconv: url = string.Format(BaiduConstParams.GeoconvApi + "?{0}", param); break;
} CurrentRequest = url; action(webClient.DownloadString(url)); }
/// /// 响应 /// /// /// /// /// public T Response(string param, BaiduLbsType baiduLbsType, Encoding encoding) { T t = default(T);
Request(param, baiduLbsType, encoding, json => { if (baiduLbsType == BaiduLbsType.GeocodingApIv2 || baiduLbsType == BaiduLbsType.GeocodingApIv2Reverse) { if (json.Contains("\"result\":[]")) { json = json.Replace("\"result\":[]", "\"result\":{}"); } } t = (T)JsonConvert.DeserializeObject(json, typeof(T)); }); return t; }
public BaiduGeocodingResults BaiduGeocoding(string address, string city) { address = System.Web.HttpUtility.UrlEncode(address); city = System.Web.HttpUtility.UrlEncode(city); string request = string.Format("address={0}&output=json&ak={1}&city={2}", address, _key, city); var result = Response(request, BaiduLbsType.GeocodingApIv2, Encoding.UTF8); if (result.Status == Status.Ok && result.Result.Location == null) { request = string.Format("address={0}&output=json&ak={1}&city={2}", city + address, _key, city); return Response(request, BaiduLbsType.GeocodingApIv2, Encoding.UTF8); } return result; }
public BaiduGeocodingResults BaiduGeocoding(string longitude, string dimensions, string pois) { var location = longitude + "," + dimensions; string request = string.Format("ak={0}&location={1}&pois={2}", _key, location, pois); return Response(request, BaiduLbsType.GeocodingApIv2, Encoding.UTF8); }
public GeoconvResults BaiduGeoconv(GeoconvParams geoconvParams, ref List geoconvPois) { geoconvParams.Ak = _key; return Response(geoconvParams.ToString(ref geoconvPois), BaiduLbsType.Geoconv, Encoding.UTF8); }
public GeoconvResults BaiduGeoconv(GeoconvParams geoconvParams, GeoconvPOI geoconvPoi) { geoconvParams.Ak = _key; List geoconvPois = new List { geoconvPoi }; return Response(geoconvParams.ToString(ref geoconvPois), BaiduLbsType.Geoconv, Encoding.UTF8); } }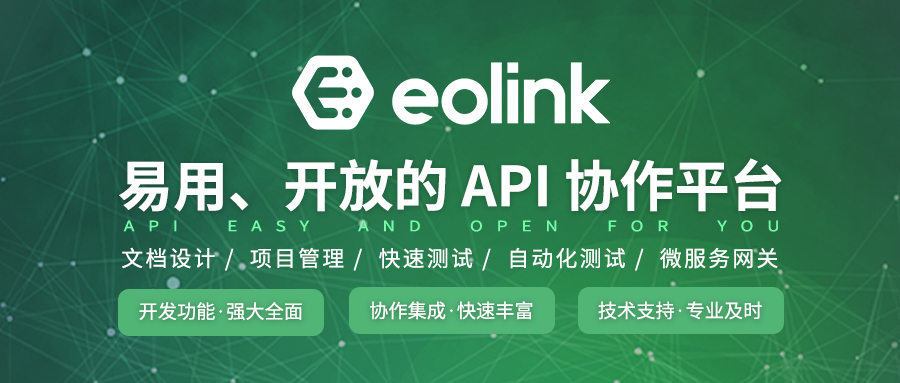
版权声明:本文内容由网络用户投稿,版权归原作者所有,本站不拥有其著作权,亦不承担相应法律责任。如果您发现本站中有涉嫌抄袭或描述失实的内容,请联系我们jiasou666@gmail.com 处理,核实后本网站将在24小时内删除侵权内容。
暂时没有评论,来抢沙发吧~