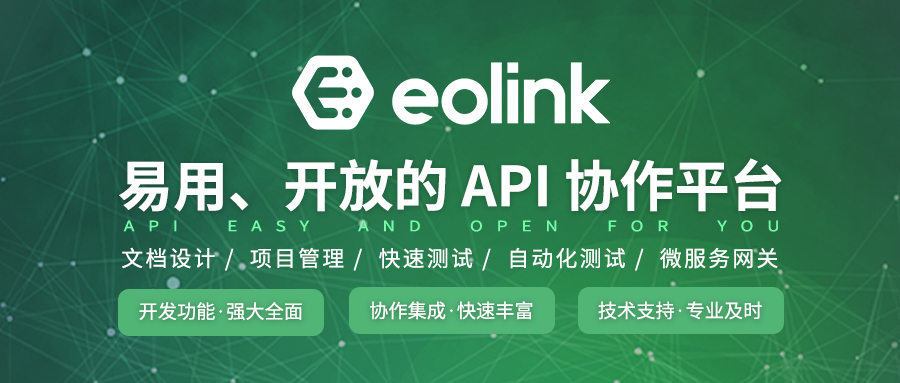
C++ map容器的简单用法
C++ map容器的简单用法
1.前言2.内容
map简介map功能map具体使用
1.构造2.增加[插入]
插入方法: insert方法 和 数组方法
3.遍历 [正向、反向、数组方法遍历]4.查找
①查找并返回key的迭代器 [find()]
备注:此处迭代器为pair对象,所以用first ,second访问
②仅判断是否存在key [count 、pair(lower_bound + upper_bound)]
5.删除 [3种]6.swap() [两种]7.排序
3.总结4.更新日志
1.前言
整理map的一些用法,欢迎指正~有具体示例解释概念,欢迎品尝~
2.内容
map简介
map是STL的关联容器,它提供一对一(其中第一个可以称为关键字,每个关键字只能在map中出现一次,第二个可能称为该关键字的值)的数据 处理能力 【key-value 】
map内部是一颗红黑树(一 种非严格意义上的平衡二叉树),这颗树具有对数据自动排序的功能
map功能
查询(log(N))、插入、删除、更改、遍历
map具体使用
1.构造
头文件: < map >示例
#include using namespace std;#include
2.增加[插入]
#include using namespace std;#include
插入方法: insert方法 和 数组方法
区别:insert不可覆盖已经插入的数据,而数组方法可以示例:
#include using namespace std;#include
3.遍历 [正向、反向、数组方法遍历]
#include using namespace std;#include
4.查找
①查找并返回key的迭代器 [find()]
备注:此处迭代器为pair对象,所以用first ,second访问
#include using namespace std;#include
②仅判断是否存在key [count 、pair(lower_bound + upper_bound)]
方法1: countmap是一对一的映射关系,则count函数返回值 只可为 0 、1,即存在返回1,不存在返回0示例:
#include using namespace std;#include
方法2:pair
前置知识:
1.equal_range() :
在[left , right)序列中表示一个数值的第一次出现与最后一次出现的后一位。得到相等元素的子范围,将两个迭代器以pair形式返回
2.lower_bound()、upper_bound()
lower_bound()返回一个 iterator 它指向在[first,last)标记的有序序列中可以插入value,而不会破坏容器顺序的第一个位置,而这个位置标记了一个不小于value 的值 即,找到>=value的位置并返回
同理,upper_bound()找到>value的位置并返回
示例
#include using namespace std;#include
正文:
#include using namespace std;#include
5.删除 [3种]
1.iterator erase(iterator it);//通过一个迭代器删除 2.iterator erase(iterator first,iterator last)//删除一个范围的元素 3.size_type erase(const Key&key); //通过关键字删除clear()就相当于Map.erase(Map.begin(),Map.end());示例:
#include using namespace std;#include
#include using namespace std;#include
6.swap() [两种]
swap的作用是,交换两个容器内的所有元素
#include using namespace std;#include
其实直接用swap()是一样的
如果用map函数可以实现的功能,而STL Algorithm也可以完成该功能,建议用map自带函数,效率高一些。
7.排序
默认按key升序排序,不可使用sort
当,关键字为 结构体时,insert等会通不过, 此时要重载 < 号
如果不重载<号,VS2019会报以下错误:
重载 <
#include using namespace std;#include
暂时没有评论,来抢沙发吧~